
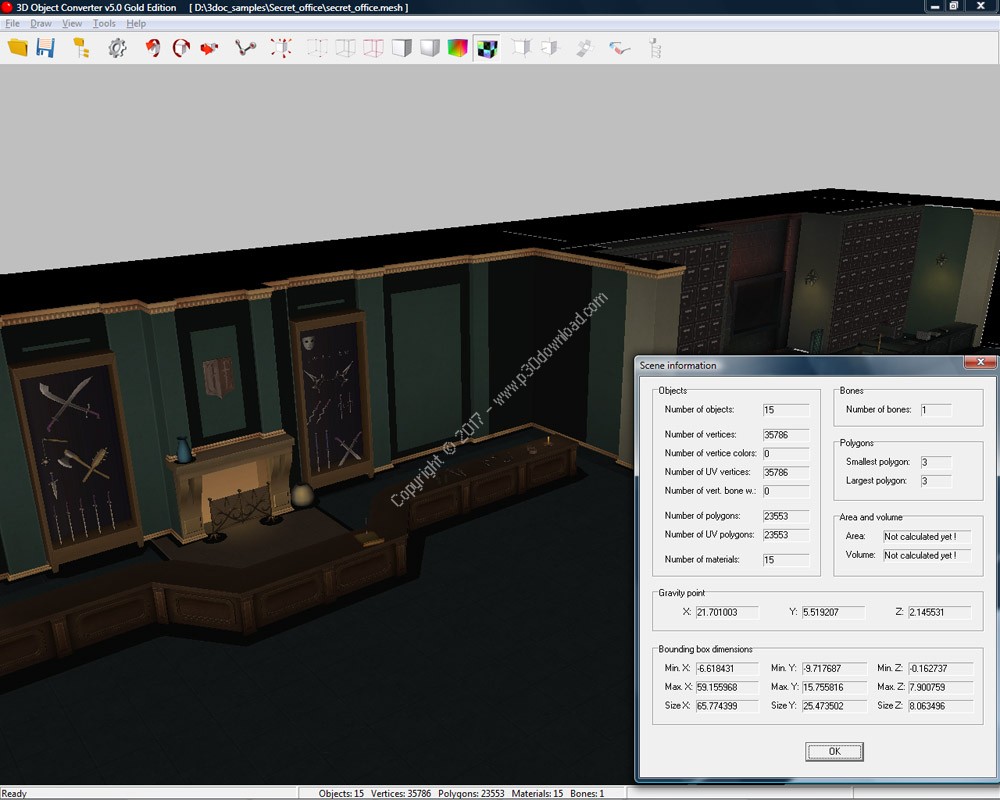
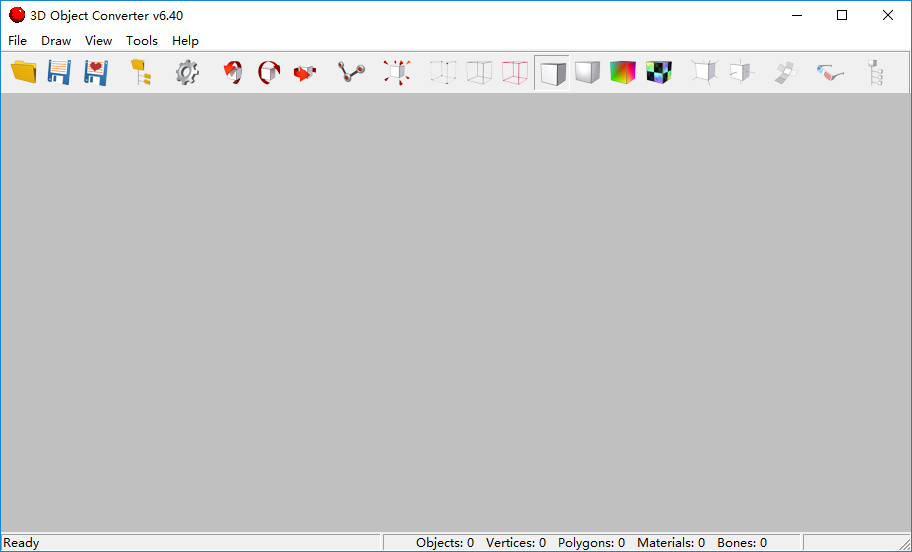
Since you are maintaining these transformations in parallel, you don't actually need to maintain the OpenGL modelview matrix anymore. You can then use your model matrix to get coordinates into world space (make sure they are homogenous coordinates first just set w = 1, if they aren't): V' = V * M

For instance, every time you call glTranslate, you would update your model matrix: T = The model matrix will contain all the transformations needed to change model coordinates into world coordinates. You need to maintain a 4x4 model matrix for each object in your scene. You can see what the transformation matrices are in the OpenGL 2.1 reference pages. Each of these functions just creates a 4x4 matrix the implements that specific transformation, then multiplies the current matrix by it. You apply transformations to the current matrix with functions like glTranslatef, glRotatef, or gluProjection. You replace the current matrix with the identity matrix using glLoadIdentity. When you call glMatrixMode, you are switching between these matrices. There is also a texture matrix we won't worry about. OpenGL internally maintains two matrices: modelview (model and view multiplied together), and projection. You would project a coordinate C onto the screen using the formula: C' = P * V * M * C
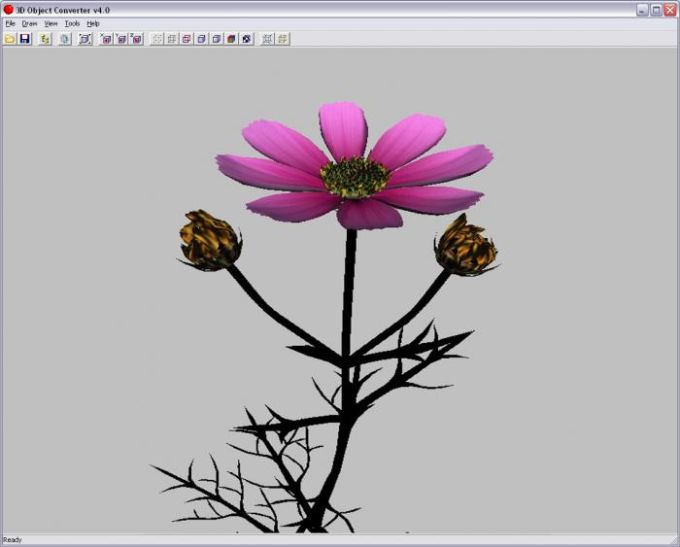
The scaling factor is needed for certain transformations like perspective projection that wouldn't be possible with non-homogenous coordinates.Ĥx4 matrices are needed to transform coordinates from one vector space to another. You can obtain coordinates in 3-space as (x/w, y/w, z/w). Projection space - everything on the screen fits in the interval in each dimension.Ĭoordinates are specified homogeneously, so each vector has components (x, y, z, w), where w is a scaling factor.View space - coordinates are specified with respect to the camera.World space - coordinates are specified with respect to some central point in the world.Model space - these are usually the coordinates you specify to OpenGL.In 3D graphics, you need to worry about several vector spaces:
